

Let's get acquainted! I'm Igor, and I am a cross-platform mobile application developer at Loovatech. In this article we are going to dive into NativeScript and its main pros and cons.
I believe my experience will benefit both developers who already write applications in React Native, Ionic, and Framework7 and those who only plan to dive into the development of cross-platform mobile applications in JavaScript.
My cross-platform development journey started outside of Loovatech - with Cordova + Framework7.
Similar to Ionic, Framework7 is simply a UI library used to create an interface, which is then rendered via WebView. The application (communication with the platform’s API) is based on Cordova or Capacitor - they also create the screen with WebView and send HTML to it.
Framework7 works great with Vue, though it has begun to bugger me off for a number of reasons. My main goal was to find a tool specifically for native development instead of technology based on WebView.
That's when I started looking towards Angular and explore different technologies available on the market for developing native cross-platform apps with Angular under the hood. That's how I got acquainted with NativeScript.


What is NativeScript
NativeScript (NS) is a platform for creating native cross-platform mobile applications in JavaScript. This platform allows you to directly access iOS/Android APIs from JavaScript, bypassing a layer of third-party plugins.
I’d say this is one of the key benefits over its older brother, React Native. Although NativeScript does not allow you to develop desktop versions of applications like Flutter and React Native, the team is already working on it. And the feature might be available some day soon.
In contrast to React Native, which only runs on React, NativeScript allows you to use Angular, Vue, React, Svelte, or vanilla JS/TS.
Cross-platform development
The cross-platform development camp could be divided into two parts: those who want to save resources and those who do not have them.
The first reason to choose cross-platform development is to get two results for less than twice the price of one. For example, a Flutter developer could build an application simultaneously for iOS and Android, and even for the web (though for now only for a somewhat undemanding customer in regards to the looks of the web part).
One C# developer can create two applications at once using Xamarin, making application development cheaper and faster. The result is visible simultaneously on all platforms, and the application looks more or less the same, so if this meets the goals of the project - great.
The second reason is that the team already has a JavaScript/TypeScript developer. A developer working with React/Vue.js/Angular can create a good-looking application, sometimes even reusing pieces of logic from an existing web app.
In my case, both factors played a role. At the start of the project, the team had front-end developers, but there were no realistic deadlines and budgets.
Since our front-end team works with Vue.js, there were a few options: HTML-based Ionic, Framework7, or native-based NativeScript. Let me say right away that my heart still belongs to Angular, which is fully supported by NativeScript.
We abandoned Ionic and Framework due to the non-native behavior of the UI and a number of limitations, though it is possible to develop prototypes and deliver new features much faster on these platforms.
We have chosen NativeScript with Vue under the hood. After a couple of years of development in this combination, we had mixed feelings that I want to share.
To immediately convince readers of the benefits of NativeScript, I'll start with its cons:
1. Very small community. The active users of the NativeScript server on Discord can be counted on one’s fingers. NativeScript's market share fell from 11% to 3% from 2019 to 2022.
2. A measly amount of third-party plugins compared to React Native, while many existing ones have not been supported for some time;
3. Even small breakdowns lead to troubleshooting the code or even your runtime environment for a long time due to the small user base. There are often cases when a project simply stops building with an esoteric error, with no changes to the code and without updating the environment - "on its own." The platform's native tools don't always directly show where the error occurred in the underlying process and sometimes simply hide it.
Well, that's probably all!
What benefits and killer features do we have?
I would list three main killer features:
1. NativeScript works with the main JS frameworks (Angular, Vue, React, Svelte) and without a framework at all;
2. Direct access to iOS and Android APIs from JavaScript/TypeScript without boilerplate - just request and use;
3. Extremely energetic, communicative, and active nuclear team. They have their own Discord, where they are always ready to discuss any question or request. For example, many of the things I requested became real features of the framework - quickly and with feedback from the team.

Let's go into more details.
JS framework support
NativeScript supports major frameworks (Angular, Vue, React, Svelte), Vanilla JS, or TS (for those who do not accept frameworks at all).
Angular and Vanilla have been supported since the introduction of NativeScript, so they have the largest number of examples and questions on Stack Overflow. After that, the platform started to support Vue, React, and Svelte. In my opinion, NativeScript applications are best made with Angular or pure TypeScript.
Mobile application development is a little more than just sending data from the server to the user's screen, like on the web. Here, you have several types of storage, working with files, phone hardware, and the network.
Using Vue complicates development a little bit. The main reason for that is that a Vuex layer is added, and we "request" our services through Vuex.
In addition, with Vue, you have to invent the project architecture yourself, and the end result often radically differs from project to project.
On the other hand, Angular offers a ready-made, well-thought-out project structure, its own DI, and full support for TypeScript and RxJs, which is more than enough when developing a mobile app. With vanilla TypeScript, you can also compose something along these lines.
Communication with the core team: a life story
In one personal project, I needed a swipe for a list item, like in a Telegram or WhatsApp chat list. The platform offered one component for lists with support for swipes (https://v7.docs.nativescript.org/ui/components/radlistview/overview), but the animation was not what I would like to see in the project.

I had to create my own component, which I later showed to the main NativeScript developer, Nathan Walker.

There really is no alternative to the RadListView component (link above), so that is something that needs to be done. Within a few months, Nathan published an article explaining how to implement swipes in CollectionView for iOS and then for Android.
The guys from the NativeScript team are always happy to answer questions on Discord. In fact, they also have "Office Hours" every month on the 4th. The team talks about the NativeScript development process and answers questions that can be asked during the stream.
Platform API access
NativeScript allows you to access the platform directly from JS, and this is something I really like about it. That is because it makes it possible to work with the platform without knowing Java, Kotlin, Swift, and, especially, Objective-C.
Below, we will look at a simple code and how a js developer can approach it. The example is taken from the official website.
Let's say there is a task to find out the battery level. We go to Google and find the documentation https://developer.apple.com/documentation/uikit/uidevice.
Here, we see the UIDevice class, which allows you to obtain information on a specific device.
Next, we go to our VS Code editor to the component class and start typing UIDevice. The editor shows us which methods are contained in the UIDevice class.

We also see all declared classes, methods, and properties for a specific platform.
Below is a fully working code to access the battery level of an iOS/Android device without writing a single line in Swift or Java.
// iOS
const formatMessage = level => `The Battery Level is: ${level}%`;
const value = UIDevice.currentDevice.batteryLevel * 100;
alert(formatMessage(value));
// Android
const formatMessage = level => `The Battery Level is: ${level}%`;
const value = bm.getIntProperty(BatteryManager.BATTERY_PROPERTY_CAPACITY);
alert(formatMessage(value));
Now, let's look at a more complex problem. We will write a mini-plugin to work with the SDK of the OneSignal service for Android and try to use its methods.
Create directory plugins/onesignal in the root with the following contents:

We are interested here in plugins/onesignal/platforms/android/include.gradle and plugins/onesignal/index.android.ts - this is what will work on Android.
// include.gradle
dependencies {
implementation 'com.onesignal:OneSignal:[5.0.0, 5.99.99]'
}
// index.android.ts
import { Utils } from '@nativescript/core';
declare const com: any;
export class OneSignal {
// основная магиях происходит в этой строке
private _oneSignal = com.onesignal.OneSignal;
static init(): void {
this._oneSignal.initWithContext(Utils.android.getApplicationContext(),
'opensignal_app_id');
}
}
Now, we can work with the OneSignal class of the com.onesignal package from JavaScript. This is what we do in the init() method.
Let's get back to the negative side of NativeScript 🙁
Very small community. The core team is made up of less than ten people. There is usually one person supporting each JS platform (NativeScript, Vue, or NativeScript React). Vue is supported by rigor789, React by shirakaba. The community is very friendly and active. Although there aren't plenty of video tutorials, examples, and solutions on YouTube, there are paid and free official video courses.
Small set of plugins. The main plugins are there, and they are supported. For example, camera, Firebase, Couchbase, SQLite, biometrics, accepting payments, etc. The list of official plugins can be found at https://docs.nativescript.org/plugins/. But OneSignal, for example, stopped being updated a long time ago. Though we have our magic API access, and we just add the library to app.gradle, write some JavaScript, and voila!
In general, there are few plugins, but they can be easily created.
Something breaks from time to time. Hot reloads sometimes fail in projects using Vue NativeScript and Angular NativeScrip. You have to shut down the process and restart.
Errors occur at the slightest update of any package; the project simply does not start - but this is a common problem with npm projects.
Peculiarities. Recommendations. Lifehacks
We are very close to the native UI elements and the API of the platform itself when developing using NativeScript. You will have to delve into native development, want it or not - at least on one platform, and at least some extent. You have to figure out the names of the elements, what properties they have, and so forth. This will allow you to get a feeling of what is happening in your application.
I recommend checking the code of the NativeScript developers when developing a plugin or when working with the native API. This helps you hone in your skills faster.
And an important thought to wrap it all up. The project, which yesterday consisted of three screens, tends to grow little by little by the creator to a size where completely different things start to matter. NativeScript does not have as wide of a support base as React Native. However, since it is simple enough, a front-end developer can work with it without special training, and due to its architecture, NativeScript allows you to succeed in even extensive and complex projects.
Useful resources:
Let's get acquainted! I'm Igor, and I am a cross-platform mobile application developer at Loovatech. In this article we are going to dive into NativeScript and its main pros and cons.
I believe my experience will benefit both developers who already write applications in React Native, Ionic, and Framework7 and those who only plan to dive into the development of cross-platform mobile applications in JavaScript.
My cross-platform development journey started outside of Loovatech - with Cordova + Framework7.
Similar to Ionic, Framework7 is simply a UI library used to create an interface, which is then rendered via WebView. The application (communication with the platform’s API) is based on Cordova or Capacitor - they also create the screen with WebView and send HTML to it.
Framework7 works great with Vue, though it has begun to bugger me off for a number of reasons. My main goal was to find a tool specifically for native development instead of technology based on WebView.
That's when I started looking towards Angular and explore different technologies available on the market for developing native cross-platform apps with Angular under the hood. That's how I got acquainted with NativeScript.


What is NativeScript
NativeScript (NS) is a platform for creating native cross-platform mobile applications in JavaScript. This platform allows you to directly access iOS/Android APIs from JavaScript, bypassing a layer of third-party plugins.
I’d say this is one of the key benefits over its older brother, React Native. Although NativeScript does not allow you to develop desktop versions of applications like Flutter and React Native, the team is already working on it. And the feature might be available some day soon.
In contrast to React Native, which only runs on React, NativeScript allows you to use Angular, Vue, React, Svelte, or vanilla JS/TS.
Cross-platform development
The cross-platform development camp could be divided into two parts: those who want to save resources and those who do not have them.
The first reason to choose cross-platform development is to get two results for less than twice the price of one. For example, a Flutter developer could build an application simultaneously for iOS and Android, and even for the web (though for now only for a somewhat undemanding customer in regards to the looks of the web part).
One C# developer can create two applications at once using Xamarin, making application development cheaper and faster. The result is visible simultaneously on all platforms, and the application looks more or less the same, so if this meets the goals of the project - great.
The second reason is that the team already has a JavaScript/TypeScript developer. A developer working with React/Vue.js/Angular can create a good-looking application, sometimes even reusing pieces of logic from an existing web app.
In my case, both factors played a role. At the start of the project, the team had front-end developers, but there were no realistic deadlines and budgets.
Since our front-end team works with Vue.js, there were a few options: HTML-based Ionic, Framework7, or native-based NativeScript. Let me say right away that my heart still belongs to Angular, which is fully supported by NativeScript.
We abandoned Ionic and Framework due to the non-native behavior of the UI and a number of limitations, though it is possible to develop prototypes and deliver new features much faster on these platforms.
We have chosen NativeScript with Vue under the hood. After a couple of years of development in this combination, we had mixed feelings that I want to share.
To immediately convince readers of the benefits of NativeScript, I'll start with its cons:
1. Very small community. The active users of the NativeScript server on Discord can be counted on one’s fingers. NativeScript's market share fell from 11% to 3% from 2019 to 2022.
2. A measly amount of third-party plugins compared to React Native, while many existing ones have not been supported for some time;
3. Even small breakdowns lead to troubleshooting the code or even your runtime environment for a long time due to the small user base. There are often cases when a project simply stops building with an esoteric error, with no changes to the code and without updating the environment - "on its own." The platform's native tools don't always directly show where the error occurred in the underlying process and sometimes simply hide it.
Well, that's probably all!
What benefits and killer features do we have?
I would list three main killer features:
1. NativeScript works with the main JS frameworks (Angular, Vue, React, Svelte) and without a framework at all;
2. Direct access to iOS and Android APIs from JavaScript/TypeScript without boilerplate - just request and use;
3. Extremely energetic, communicative, and active nuclear team. They have their own Discord, where they are always ready to discuss any question or request. For example, many of the things I requested became real features of the framework - quickly and with feedback from the team.

Let's go into more details.
JS framework support
NativeScript supports major frameworks (Angular, Vue, React, Svelte), Vanilla JS, or TS (for those who do not accept frameworks at all).
Angular and Vanilla have been supported since the introduction of NativeScript, so they have the largest number of examples and questions on Stack Overflow. After that, the platform started to support Vue, React, and Svelte. In my opinion, NativeScript applications are best made with Angular or pure TypeScript.
Mobile application development is a little more than just sending data from the server to the user's screen, like on the web. Here, you have several types of storage, working with files, phone hardware, and the network.
Using Vue complicates development a little bit. The main reason for that is that a Vuex layer is added, and we "request" our services through Vuex.
In addition, with Vue, you have to invent the project architecture yourself, and the end result often radically differs from project to project.
On the other hand, Angular offers a ready-made, well-thought-out project structure, its own DI, and full support for TypeScript and RxJs, which is more than enough when developing a mobile app. With vanilla TypeScript, you can also compose something along these lines.
Communication with the core team: a life story
In one personal project, I needed a swipe for a list item, like in a Telegram or WhatsApp chat list. The platform offered one component for lists with support for swipes (https://v7.docs.nativescript.org/ui/components/radlistview/overview), but the animation was not what I would like to see in the project.

I had to create my own component, which I later showed to the main NativeScript developer, Nathan Walker.

There really is no alternative to the RadListView component (link above), so that is something that needs to be done. Within a few months, Nathan published an article explaining how to implement swipes in CollectionView for iOS and then for Android.
The guys from the NativeScript team are always happy to answer questions on Discord. In fact, they also have "Office Hours" every month on the 4th. The team talks about the NativeScript development process and answers questions that can be asked during the stream.
Platform API access
NativeScript allows you to access the platform directly from JS, and this is something I really like about it. That is because it makes it possible to work with the platform without knowing Java, Kotlin, Swift, and, especially, Objective-C.
Below, we will look at a simple code and how a js developer can approach it. The example is taken from the official website.
Let's say there is a task to find out the battery level. We go to Google and find the documentation https://developer.apple.com/documentation/uikit/uidevice.
Here, we see the UIDevice class, which allows you to obtain information on a specific device.
Next, we go to our VS Code editor to the component class and start typing UIDevice. The editor shows us which methods are contained in the UIDevice class.

We also see all declared classes, methods, and properties for a specific platform.
Below is a fully working code to access the battery level of an iOS/Android device without writing a single line in Swift or Java.
// iOS
const formatMessage = level => `The Battery Level is: ${level}%`;
const value = UIDevice.currentDevice.batteryLevel * 100;
alert(formatMessage(value));
// Android
const formatMessage = level => `The Battery Level is: ${level}%`;
const value = bm.getIntProperty(BatteryManager.BATTERY_PROPERTY_CAPACITY);
alert(formatMessage(value));
Now, let's look at a more complex problem. We will write a mini-plugin to work with the SDK of the OneSignal service for Android and try to use its methods.
Create directory plugins/onesignal in the root with the following contents:

We are interested here in plugins/onesignal/platforms/android/include.gradle and plugins/onesignal/index.android.ts - this is what will work on Android.
// include.gradle
dependencies {
implementation 'com.onesignal:OneSignal:[5.0.0, 5.99.99]'
}
// index.android.ts
import { Utils } from '@nativescript/core';
declare const com: any;
export class OneSignal {
// основная магиях происходит в этой строке
private _oneSignal = com.onesignal.OneSignal;
static init(): void {
this._oneSignal.initWithContext(Utils.android.getApplicationContext(),
'opensignal_app_id');
}
}
Now, we can work with the OneSignal class of the com.onesignal package from JavaScript. This is what we do in the init() method.
Let's get back to the negative side of NativeScript 🙁
Very small community. The core team is made up of less than ten people. There is usually one person supporting each JS platform (NativeScript, Vue, or NativeScript React). Vue is supported by rigor789, React by shirakaba. The community is very friendly and active. Although there aren't plenty of video tutorials, examples, and solutions on YouTube, there are paid and free official video courses.
Small set of plugins. The main plugins are there, and they are supported. For example, camera, Firebase, Couchbase, SQLite, biometrics, accepting payments, etc. The list of official plugins can be found at https://docs.nativescript.org/plugins/. But OneSignal, for example, stopped being updated a long time ago. Though we have our magic API access, and we just add the library to app.gradle, write some JavaScript, and voila!
In general, there are few plugins, but they can be easily created.
Something breaks from time to time. Hot reloads sometimes fail in projects using Vue NativeScript and Angular NativeScrip. You have to shut down the process and restart.
Errors occur at the slightest update of any package; the project simply does not start - but this is a common problem with npm projects.
Peculiarities. Recommendations. Lifehacks
We are very close to the native UI elements and the API of the platform itself when developing using NativeScript. You will have to delve into native development, want it or not - at least on one platform, and at least some extent. You have to figure out the names of the elements, what properties they have, and so forth. This will allow you to get a feeling of what is happening in your application.
I recommend checking the code of the NativeScript developers when developing a plugin or when working with the native API. This helps you hone in your skills faster.
And an important thought to wrap it all up. The project, which yesterday consisted of three screens, tends to grow little by little by the creator to a size where completely different things start to matter. NativeScript does not have as wide of a support base as React Native. However, since it is simple enough, a front-end developer can work with it without special training, and due to its architecture, NativeScript allows you to succeed in even extensive and complex projects.
Useful resources:
Let's get acquainted! I'm Igor, and I am a cross-platform mobile application developer at Loovatech. In this article we are going to dive into NativeScript and its main pros and cons.
I believe my experience will benefit both developers who already write applications in React Native, Ionic, and Framework7 and those who only plan to dive into the development of cross-platform mobile applications in JavaScript.
My cross-platform development journey started outside of Loovatech - with Cordova + Framework7.
Similar to Ionic, Framework7 is simply a UI library used to create an interface, which is then rendered via WebView. The application (communication with the platform’s API) is based on Cordova or Capacitor - they also create the screen with WebView and send HTML to it.
Framework7 works great with Vue, though it has begun to bugger me off for a number of reasons. My main goal was to find a tool specifically for native development instead of technology based on WebView.
That's when I started looking towards Angular and explore different technologies available on the market for developing native cross-platform apps with Angular under the hood. That's how I got acquainted with NativeScript.


What is NativeScript
NativeScript (NS) is a platform for creating native cross-platform mobile applications in JavaScript. This platform allows you to directly access iOS/Android APIs from JavaScript, bypassing a layer of third-party plugins.
I’d say this is one of the key benefits over its older brother, React Native. Although NativeScript does not allow you to develop desktop versions of applications like Flutter and React Native, the team is already working on it. And the feature might be available some day soon.
In contrast to React Native, which only runs on React, NativeScript allows you to use Angular, Vue, React, Svelte, or vanilla JS/TS.
Cross-platform development
The cross-platform development camp could be divided into two parts: those who want to save resources and those who do not have them.
The first reason to choose cross-platform development is to get two results for less than twice the price of one. For example, a Flutter developer could build an application simultaneously for iOS and Android, and even for the web (though for now only for a somewhat undemanding customer in regards to the looks of the web part).
One C# developer can create two applications at once using Xamarin, making application development cheaper and faster. The result is visible simultaneously on all platforms, and the application looks more or less the same, so if this meets the goals of the project - great.
The second reason is that the team already has a JavaScript/TypeScript developer. A developer working with React/Vue.js/Angular can create a good-looking application, sometimes even reusing pieces of logic from an existing web app.
In my case, both factors played a role. At the start of the project, the team had front-end developers, but there were no realistic deadlines and budgets.
Since our front-end team works with Vue.js, there were a few options: HTML-based Ionic, Framework7, or native-based NativeScript. Let me say right away that my heart still belongs to Angular, which is fully supported by NativeScript.
We abandoned Ionic and Framework due to the non-native behavior of the UI and a number of limitations, though it is possible to develop prototypes and deliver new features much faster on these platforms.
We have chosen NativeScript with Vue under the hood. After a couple of years of development in this combination, we had mixed feelings that I want to share.
To immediately convince readers of the benefits of NativeScript, I'll start with its cons:
1. Very small community. The active users of the NativeScript server on Discord can be counted on one’s fingers. NativeScript's market share fell from 11% to 3% from 2019 to 2022.
2. A measly amount of third-party plugins compared to React Native, while many existing ones have not been supported for some time;
3. Even small breakdowns lead to troubleshooting the code or even your runtime environment for a long time due to the small user base. There are often cases when a project simply stops building with an esoteric error, with no changes to the code and without updating the environment - "on its own." The platform's native tools don't always directly show where the error occurred in the underlying process and sometimes simply hide it.
Well, that's probably all!
What benefits and killer features do we have?
I would list three main killer features:
1. NativeScript works with the main JS frameworks (Angular, Vue, React, Svelte) and without a framework at all;
2. Direct access to iOS and Android APIs from JavaScript/TypeScript without boilerplate - just request and use;
3. Extremely energetic, communicative, and active nuclear team. They have their own Discord, where they are always ready to discuss any question or request. For example, many of the things I requested became real features of the framework - quickly and with feedback from the team.

Let's go into more details.
JS framework support
NativeScript supports major frameworks (Angular, Vue, React, Svelte), Vanilla JS, or TS (for those who do not accept frameworks at all).
Angular and Vanilla have been supported since the introduction of NativeScript, so they have the largest number of examples and questions on Stack Overflow. After that, the platform started to support Vue, React, and Svelte. In my opinion, NativeScript applications are best made with Angular or pure TypeScript.
Mobile application development is a little more than just sending data from the server to the user's screen, like on the web. Here, you have several types of storage, working with files, phone hardware, and the network.
Using Vue complicates development a little bit. The main reason for that is that a Vuex layer is added, and we "request" our services through Vuex.
In addition, with Vue, you have to invent the project architecture yourself, and the end result often radically differs from project to project.
On the other hand, Angular offers a ready-made, well-thought-out project structure, its own DI, and full support for TypeScript and RxJs, which is more than enough when developing a mobile app. With vanilla TypeScript, you can also compose something along these lines.
Communication with the core team: a life story
In one personal project, I needed a swipe for a list item, like in a Telegram or WhatsApp chat list. The platform offered one component for lists with support for swipes (https://v7.docs.nativescript.org/ui/components/radlistview/overview), but the animation was not what I would like to see in the project.

I had to create my own component, which I later showed to the main NativeScript developer, Nathan Walker.

There really is no alternative to the RadListView component (link above), so that is something that needs to be done. Within a few months, Nathan published an article explaining how to implement swipes in CollectionView for iOS and then for Android.
The guys from the NativeScript team are always happy to answer questions on Discord. In fact, they also have "Office Hours" every month on the 4th. The team talks about the NativeScript development process and answers questions that can be asked during the stream.
Platform API access
NativeScript allows you to access the platform directly from JS, and this is something I really like about it. That is because it makes it possible to work with the platform without knowing Java, Kotlin, Swift, and, especially, Objective-C.
Below, we will look at a simple code and how a js developer can approach it. The example is taken from the official website.
Let's say there is a task to find out the battery level. We go to Google and find the documentation https://developer.apple.com/documentation/uikit/uidevice.
Here, we see the UIDevice class, which allows you to obtain information on a specific device.
Next, we go to our VS Code editor to the component class and start typing UIDevice. The editor shows us which methods are contained in the UIDevice class.

We also see all declared classes, methods, and properties for a specific platform.
Below is a fully working code to access the battery level of an iOS/Android device without writing a single line in Swift or Java.
// iOS
const formatMessage = level => `The Battery Level is: ${level}%`;
const value = UIDevice.currentDevice.batteryLevel * 100;
alert(formatMessage(value));
// Android
const formatMessage = level => `The Battery Level is: ${level}%`;
const value = bm.getIntProperty(BatteryManager.BATTERY_PROPERTY_CAPACITY);
alert(formatMessage(value));
Now, let's look at a more complex problem. We will write a mini-plugin to work with the SDK of the OneSignal service for Android and try to use its methods.
Create directory plugins/onesignal in the root with the following contents:

We are interested here in plugins/onesignal/platforms/android/include.gradle and plugins/onesignal/index.android.ts - this is what will work on Android.
// include.gradle
dependencies {
implementation 'com.onesignal:OneSignal:[5.0.0, 5.99.99]'
}
// index.android.ts
import { Utils } from '@nativescript/core';
declare const com: any;
export class OneSignal {
// основная магиях происходит в этой строке
private _oneSignal = com.onesignal.OneSignal;
static init(): void {
this._oneSignal.initWithContext(Utils.android.getApplicationContext(),
'opensignal_app_id');
}
}
Now, we can work with the OneSignal class of the com.onesignal package from JavaScript. This is what we do in the init() method.
Let's get back to the negative side of NativeScript 🙁
Very small community. The core team is made up of less than ten people. There is usually one person supporting each JS platform (NativeScript, Vue, or NativeScript React). Vue is supported by rigor789, React by shirakaba. The community is very friendly and active. Although there aren't plenty of video tutorials, examples, and solutions on YouTube, there are paid and free official video courses.
Small set of plugins. The main plugins are there, and they are supported. For example, camera, Firebase, Couchbase, SQLite, biometrics, accepting payments, etc. The list of official plugins can be found at https://docs.nativescript.org/plugins/. But OneSignal, for example, stopped being updated a long time ago. Though we have our magic API access, and we just add the library to app.gradle, write some JavaScript, and voila!
In general, there are few plugins, but they can be easily created.
Something breaks from time to time. Hot reloads sometimes fail in projects using Vue NativeScript and Angular NativeScrip. You have to shut down the process and restart.
Errors occur at the slightest update of any package; the project simply does not start - but this is a common problem with npm projects.
Peculiarities. Recommendations. Lifehacks
We are very close to the native UI elements and the API of the platform itself when developing using NativeScript. You will have to delve into native development, want it or not - at least on one platform, and at least some extent. You have to figure out the names of the elements, what properties they have, and so forth. This will allow you to get a feeling of what is happening in your application.
I recommend checking the code of the NativeScript developers when developing a plugin or when working with the native API. This helps you hone in your skills faster.
And an important thought to wrap it all up. The project, which yesterday consisted of three screens, tends to grow little by little by the creator to a size where completely different things start to matter. NativeScript does not have as wide of a support base as React Native. However, since it is simple enough, a front-end developer can work with it without special training, and due to its architecture, NativeScript allows you to succeed in even extensive and complex projects.
Useful resources:
let's talk
book a 30-minute call to get feedback and a budget estimate from our expert team
let's talk
book a 30-minute call to get feedback and a budget estimate from our expert team
let's talk
book a 30-minute call to get feedback and a budget estimate from our expert team
our address
Tornimäe, 5 10145 Tallinn Estonia
contact us

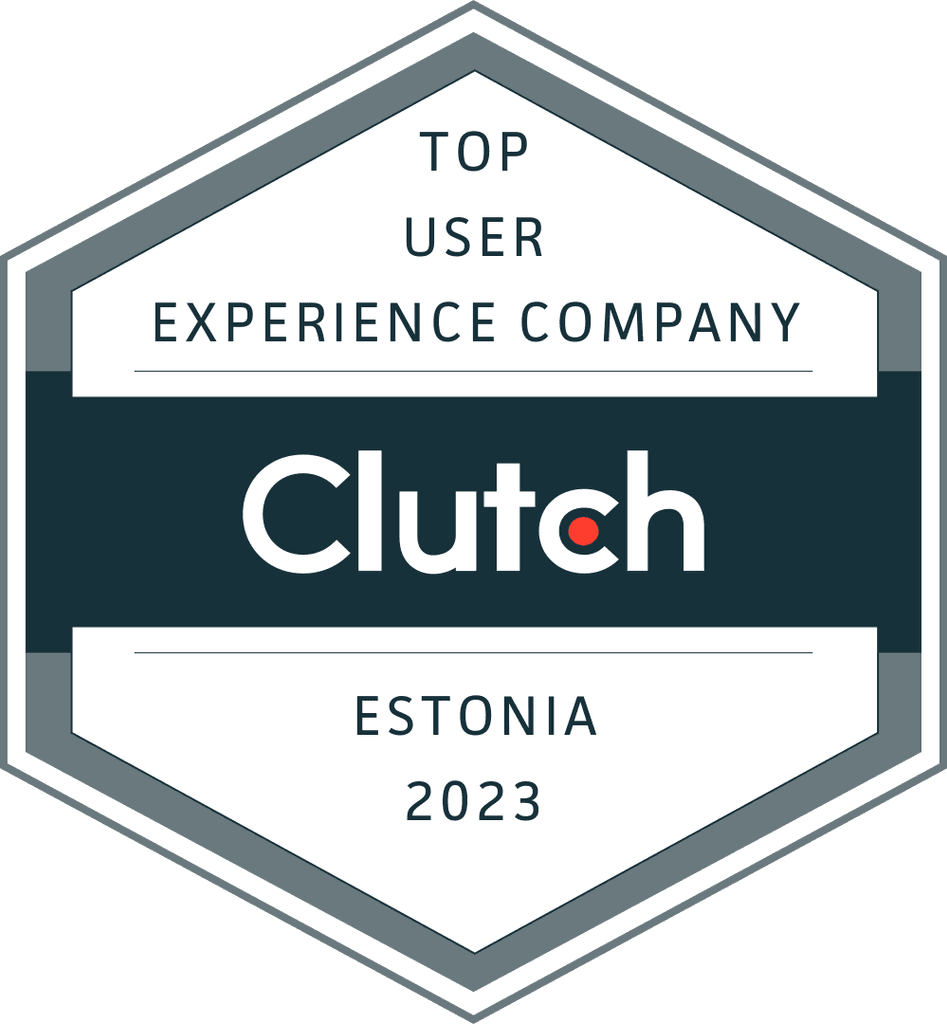
our address
Tornimäe, 5 10145 Tallinn Estonia
contact us

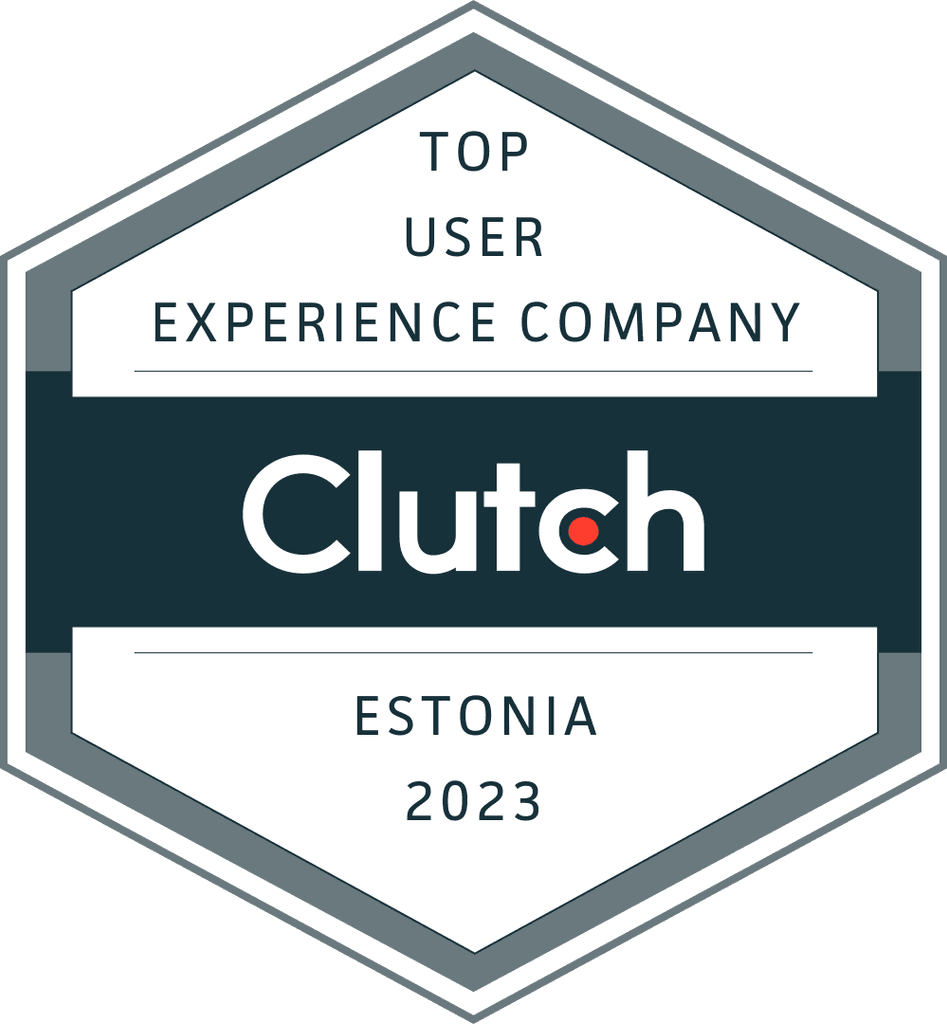
our address
Tornimäe, 5 10145 Tallinn Estonia
contact us

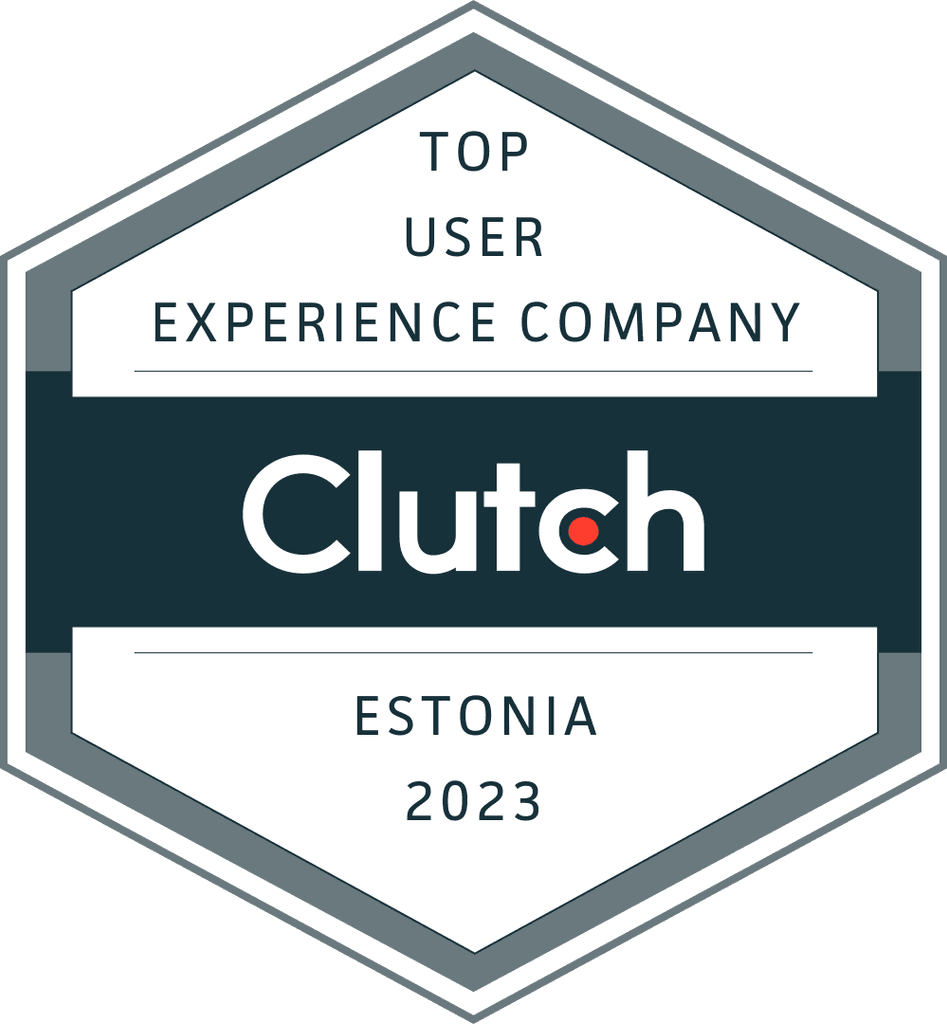